LeetCode Solutions 65
My solutions to some LeetCode-style coding problems.
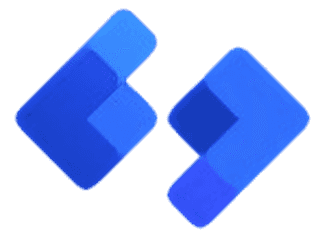
3-6-9
- python
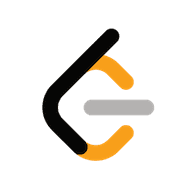
Best Time to Buy and Sell Stock
- javascript
- arrays
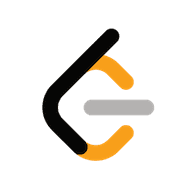
Binary Search
- python
- javascript
- binary search
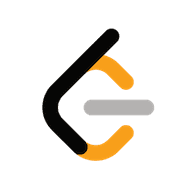
Binary Tree Inorder Traversal
- python
- binary trees
- inorder traversal
- dfs
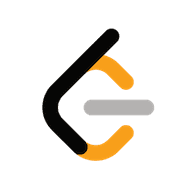
Binary Tree Postorder Traversal
- python
- binary trees
- postorder traversal
- dfs
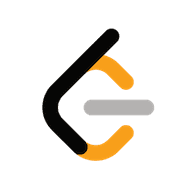
Binary Tree Preorder Traversal
- python
- binary trees
- preorder traversal
- dfs
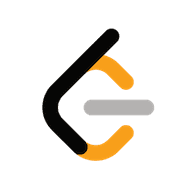
Climbing Stairs
- python
- javascript
- memoization
- dynamic programming
- fibonacci
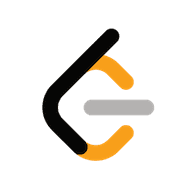
Contains Duplicate
- python
- hash table
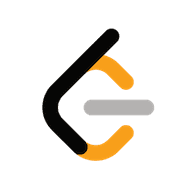
Defanging an IP Address
- javascript
- strings
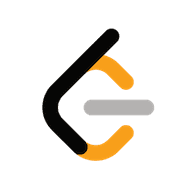
Delete Node in a Linked List
- python
- linked lists
- in-place
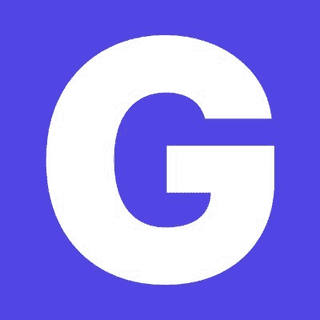
Drop Right While
- javascript
- typescript
- arrays
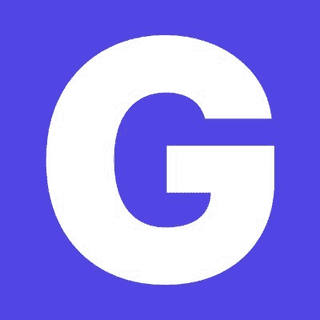
Drop While
- javascript
- typescript
- arrays
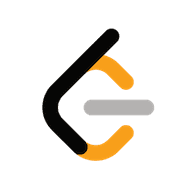
Excel Sheet Column Number
- python
- math
- strings
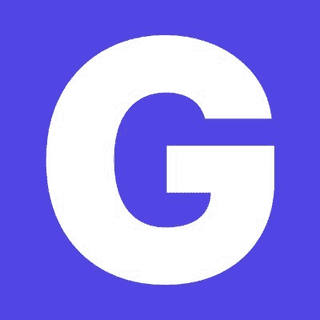
Find Index
- javascript
- typescript
- arrays
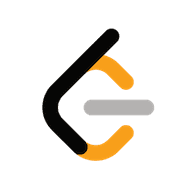
Find Pivot Index
- python
- prefix sum
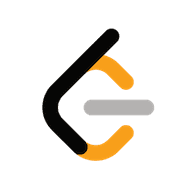
Find the Middle Index in Array
- python
- lists
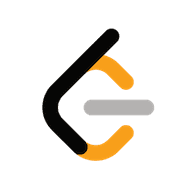
Find the Index of the First Occurrence in a String
- javascript
- strings
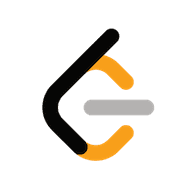
Fizz Buzz
- python
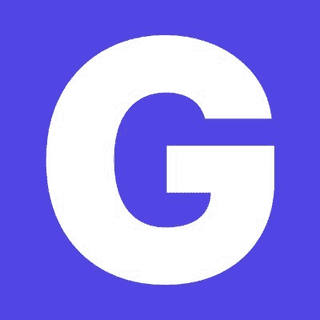
Flatten
- javascript
- arrays
- recursion
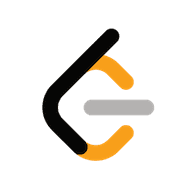
Happy Number
- python
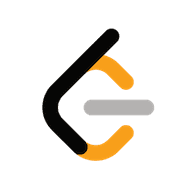
Implement Queue using Stacks
- javascript
- stack
- queue
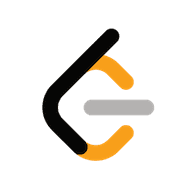
Invert Binary Tree
- javascript
- binary tree
- bfs
- dfs
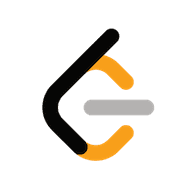
Is Subsequence
- javascript
- typescript
- string
- two pointers
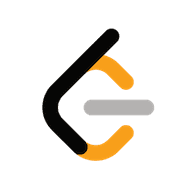
Kids With the Greatest Number of Candies
- javascript
- typescript
- array
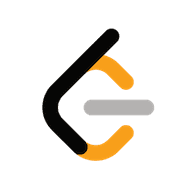
Length of Last Word
- javascript
- strings
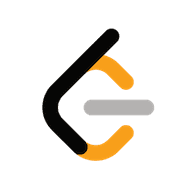
Longest Common Prefix
- python
- strings
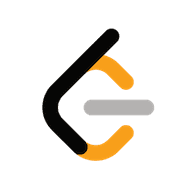
Longest Substring Without Repeating Characters
- javascript
- strings
- hash tables
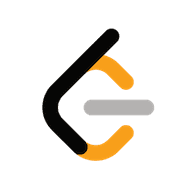
Majority Element
- python
- hash tables
- sorting
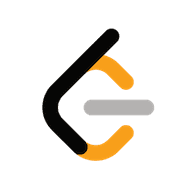
Merge Sorted Array
- javascript
- array
- sorting
- two pointers
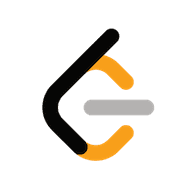
Merge Two Sorted Lists
- python
- javascript
- linked lists
- sorting
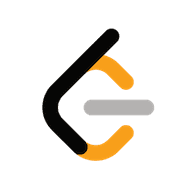
Move Zeroes
- python
- javascript
- in-place
- two pointers
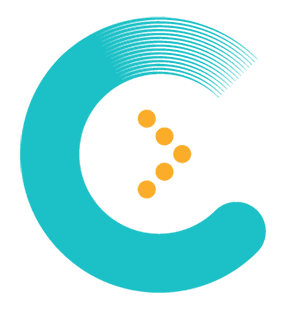
Moving Median
- javascript
- arrays
- median
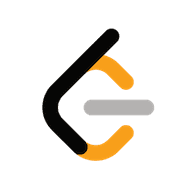
Number of Good Pairs
- javascript
- hash table
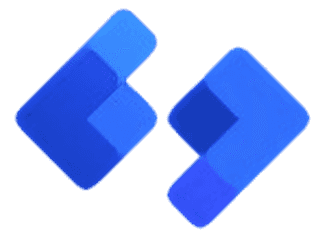
Detect the Only Duplicate in a List
- python
- two pointers
- array
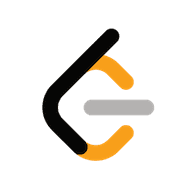
Palindrome Number
- python
- palindrome
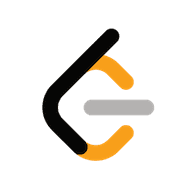
Pascal's Triangle I
- python
- arrays
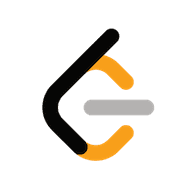
Pascal's Triangle II
- python
- arrays
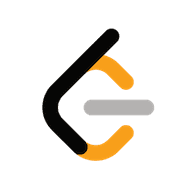
Plus One
- javascript
- array
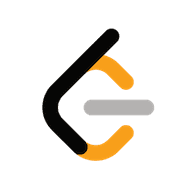
Remove Duplicates from Sorted Array
- javascript
- arrays
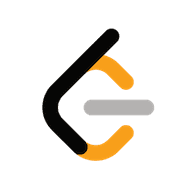
Remove Duplicates from Sorted List
- python
- data structures
- linked list
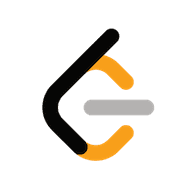
Remove Element
- javascript
- arrays
- in-place
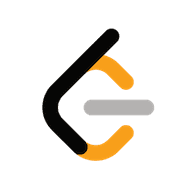
Remove Linked List Elements
- python
- linked lists
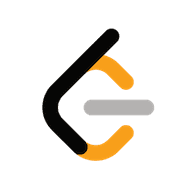
Reverse Integer
- javascript
- math
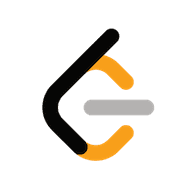
Reverse Linked List
- python
- linked lists
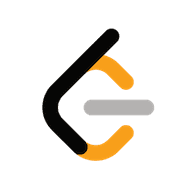
Reverse String
- python
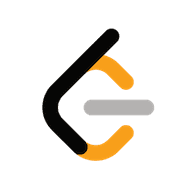
Reverse Vowels of a String
- javascript
- typescript
- string
- two pointers
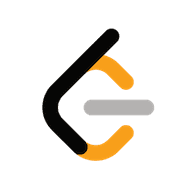
Reverse Words in a String
- javascript
- typescript
- string
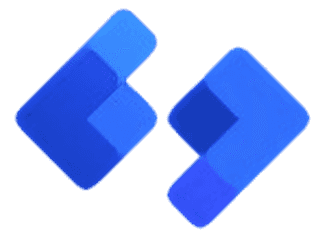
Robinhood
- python
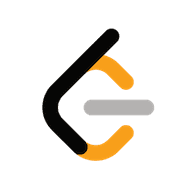
Roman to Integer
- python
- math
- numbers
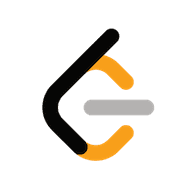
Running Sum of a 1D Array
- python
- prefix sum
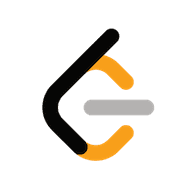
Search Insertion Position
- javascript
- arrays
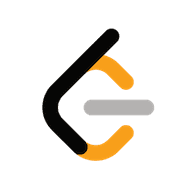
Single Number
- python
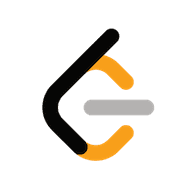
Single Number II
- python
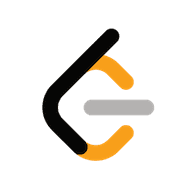
Sliding Window Median
- python
- sliding window
- arrays
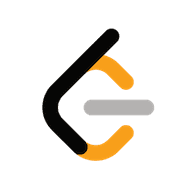
Sort Array By Parity
- javascript
- arrays
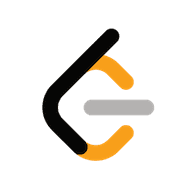
Squares of a Sorted Array
- javascript
- two pointers
- array
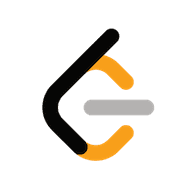
To Lower Case
- javascript
- strings
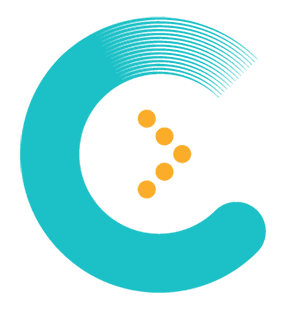
Tree Constructor
- javascript
- binary tree
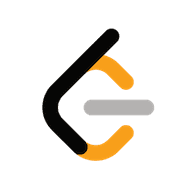
Two Sum
- python
- javascript
- typescript

Undirected Path
- python
- graph
- traversal
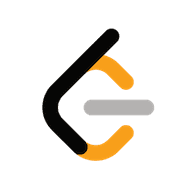
Unique Paths
- javascript
- dynamic programming
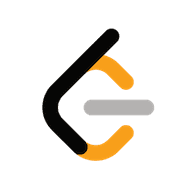
Valid Anagram
- javascript
- strings
- hash tables
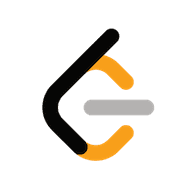
Valid Palindrome
- javascript
- python
- palindrome
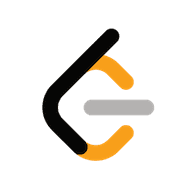
Valid Palindrome II
- javascript
- strings
- dynamic programming
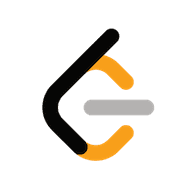
Valid Parentheses
- python
- stack